详解Python制作“坦克飞机大战”游戏
编辑日期: 2024-07-09 文章阅读: 次
2024年07月11日,第二次修订。
代码和资源文件打包文末提供下载:
详解如何制作一个简单的“坦克飞机大战”游戏。这里我们使用Python和Pygame库来开发这个游戏。如果你没有安装Pygame,可以使用以下命令安装:
pip install pygame
第一步:导入项目所需模块
项目全部模块:
import math
import random
import pygame
from pygame import mixer
第二步:项目初始化
初始化项目:
WINDOW_WIDTH, WINDOW_HEIGHT = 800, 600
TANK_INIT_POS = (370, 480)
TANK_MOVE_DELTA = 5
PLANE_MOVE_DELTA = (4, 40)
PLANE_GOAL_POS_Y = 440
PLANE_OCCUR_RANGE_Y = (50, 150)
COLL_DIST_PLANE_BULLET = 27
BULLET_FIRE_RELA_TANK = (16, 10)
pygame.init()
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("坦克飞机大战")
icon = pygame.image.load('ico.png')
pygame.display.set_icon(icon)
background = pygame.image.load('background.png')
mixer.music.load("bg.wav")
mixer.music.play(-1)
tank_img = pygame.image.load('tank.png')
tank_pos_x, tank_pos_y = TANK_INIT_POS
tank_x_change = 0
plane_imgs = []
plane_x = []
plane_y = []
plane_x_change = []
plane_y_change = []
num_of_planes = 6
for i in range(num_of_planes):
plane_img = pygame.image.load('plane.png')
plane_imgs.append(plane_img)
plane_x.append(random.randint(0, WINDOW_WIDTH - plane_img.get_rect().width))
plane_y.append(random.randint(WINDOW_HEIGHT // 12, WINDOW_HEIGHT // 2))
plane_x_change.append(PLANE_MOVE_DELTA[0])
plane_y_change.append(PLANE_MOVE_DELTA[1])
bullet_img = pygame.image.load('bullet.png')
bullet_x = 0
bullet_y = tank_pos_y
bullet_y_change = 10
bullet_state = "ready"
score_value = 0
font = pygame.font.Font(None, 32)
text_x = 10
text_y = 10
over_font = pygame.font.Font(None, 64)
第三步:定义所需函数
def show_score(x, y):
score = font.render(f"Score : {score_value}", True, (255, 255, 255))
screen.blit(score, (x, y))
def game_over_text():
over_text = over_font.render("GAME OVER", True, (255, 255, 255))
screen.blit(over_text, (200, 250))
def draw_tank(x, y):
screen.blit(tank_img, (x, y))
def draw_plane(x, y, i):
screen.blit(plane_imgs[i], (x, y))
def fire_bullet(x, y):
global bullet_state
bullet_state = "fire"
screen.blit(bullet_img, (x + BULLET_FIRE_RELA_TANK[0], y + BULLET_FIRE_RELA_TANK[1]))
def is_collision(enemy_x, enemy_y, bullet_x, bullet_y):
distance = math.sqrt(math.pow(enemy_x - bullet_x, 2) +
(math.pow(enemy_y - bullet_y, 2))
)
return distance < COLL_DIST_PLANE_BULLET
第四步:主逻辑
if __name__ == "__main__":
running = True
while running:
screen.fill((0, 0, 0))
screen.blit(background, (0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
tank_x_change = -TANK_MOVE_DELTA
if event.key == pygame.K_RIGHT:
tank_x_change = TANK_MOVE_DELTA
if event.key == pygame.K_SPACE:
if bullet_state == "ready":
mixer.Sound("laser.wav").play()
bullet_x = tank_pos_x
fire_bullet(bullet_x, bullet_y)
if event.type == pygame.KEYUP:
if event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
tank_x_change = 0
tank_pos_x += tank_x_change
if tank_pos_x <= 0:
tank_pos_x = 0
elif tank_pos_x >= WINDOW_WIDTH - plane_img.get_rect().width:
tank_pos_x = WINDOW_WIDTH - plane_img.get_rect().width
for i in range(num_of_planes):
if plane_y[i] > PLANE_GOAL_POS_Y:
for j in range(num_of_planes):
plane_y[j] = 2000
game_over_text()
break
plane_x[i] += plane_x_change[i]
if plane_x[i] <= 0:
plane_x_change[i] = PLANE_MOVE_DELTA[0]
plane_y[i] += plane_y_change[i]
elif plane_x[i] >= WINDOW_WIDTH - plane_img.get_rect().width:
plane_x_change[i] = -PLANE_MOVE_DELTA[0]
plane_y[i] += plane_y_change[i]
collision = is_collision(plane_x[i], plane_y[i], bullet_x, bullet_y)
if collision:
explosion_sound = mixer.Sound("explosion.wav")
explosion_sound.play()
bullet_y = tank_pos_y
bullet_state = "ready"
score_value += 1
plane_x[i] = random.randint(0, WINDOW_WIDTH - plane_img.get_rect().width)
plane_y[i] = random.randint(PLANE_OCCUR_RANGE_Y[0], PLANE_OCCUR_RANGE_Y[1])
draw_plane(plane_x[i], plane_y[i], i)
if bullet_y <= 0:
bullet_y = tank_pos_y
bullet_state = "ready"
if bullet_state == "fire":
fire_bullet(bullet_x, bullet_y)
bullet_y -= bullet_y_change
draw_tank(tank_pos_x, tank_pos_y)
show_score(text_x, text_y)
pygame.display.update()
以上代码完全可运行,运行后窗口:
项目完整代码,所依赖的图片和背景音乐资源文件,完整打包下载,请在我的下面公众号回复:飞机坦克
,获取全部资料:
大家在看

AI安装教程
AI本地安装教程

微软AI大模型通识教程
微软AI大模型通识教程

AI大模型入门教程
AI大模型入门教程
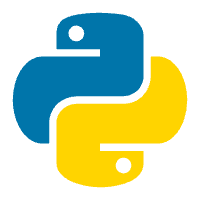
Python入门教程
Python入门教程

Python进阶教程
Python进阶教程

Python小例子200道练习题
Python小例子200道练习题

Python练手项目
Python练手项目
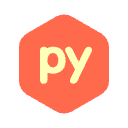
Python从零在线练习题
Python从零到一60题